sampler_deo_tempering_MCMC< S > Class Template Reference
Parallel tempered Markov Chain Monte Carlo using DEO swapping scheme. More...
#include "sampling/sampler_deo_tempering_MCMC.h"
Inheritance diagram for sampler_deo_tempering_MCMC< S >:
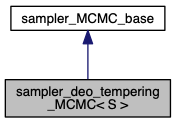
Collaboration diagram for sampler_deo_tempering_MCMC< S >:
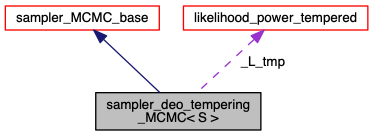
Public Member Functions | |
sampler_deo_tempering_MCMC (int seed, likelihood_power_tempered &L, std::vector< std::string > var_names, size_t dimension) | |
Class constructor, takes a reference to the exploration kernel to be used. | |
void | set_initial_location (std::vector< double > initial_parameters) |
void | run_sampler (int number_of_rounds, int thin, int refresh, int verbosity=0) |
Runs DEO using where you specify the starting run length and adaption parameters. After each round each number if increased by the geometric increase factor specified in set_annealing_schedule. More... | |
S * | get_sampler () |
Provides access to the exploration sampler so that you can change options for it. Returns a pointer to the sampler. | |
int | get_round () const |
Returns the current round of the sampler. | |
void | set_output_stream (std::string chain_file, std::string state_file, std::string sampler_file, int output_precision=8) |
Sets the output streams for the sampler. This is the same function as for sampler_MCMC_base. More... | |
void | set_deo_round_params (int initial_length, int swap_stride) |
Sets the annealing schedule to be used. More... | |
void | set_annealing_schedule (double initial_spacing, int geometric_increase=2) |
void | set_annealing_output (std::string annealing_file, std::string annealing_summary_file="") |
Sets the annealing file and summary file names. If using the default for the annealing_summary_file the output will be the annealing_file+".summary". | |
void | set_cpu_distribution (int num_temperatures, int num_exploration) |
Sets the distribution for the cpu. More... | |
void | read_initial_ladder (std::string annealing_file) |
Reads in an annealing.dat file and set the initial ladder for the sampler to the ladder from the final round in the annealing file. More... | |
void | mpi_cleanup () |
Cleans up the MPI instances of the sampler to gracefully exit;. | |
void | get_chain_state (std::vector< double > &chain, std::vector< double > &state) |
void | set_chain_state (std::vector< double > chain, std::vector< double > state) |
std::vector< double > | find_best_fit (std::string chain_file, std::string state_file) const |
Finds the best fit within provided chain file and returns it. More... | |
std::vector< double > | find_best_fit () const |
Finds the best fit from the chain and state file saved in set_output_stream. More... | |
void | write_checkpoint (std::string file) |
void | write_checkpoint (std::ostream &file) |
void | read_checkpoint (std::istream &ckpt_in) |
Loads the ckpt from a stream. This will set the sampler to the state given in the ckpt. More... | |
void | read_checkpoint (std::string ckpt_file) |
Reads the checkpoint file and restores the sampler to that state. More... | |
void | set_checkpoint (int ckpt_stride, std::string ckpt_file) |
double | estimate_bayesian_evidence (double avg_lklhd[], double beta[]) |
Function to estimate the bayesian evidence using the Thermodynamic Integration method. More... | |
![]() | |
sampler_MCMC_base (int seed, likelihood &L, std::vector< std::string > var_names, size_t dimension) | |
void | set_checkpoint (int ckpt_stride, std::string ckpt_file) |
Sets the checkpoint/restart functionality. More... | |
virtual void | reset_sampler_step () |
void | reset_likelihood_sum () |
double | get_likelihood_sum () |
virtual double | get_rand () |
virtual void | reset_rng_seed (int seed) |
void | set_mpi_communicator (MPI_Comm comm) |
Sets the MPI_Comm to be used for the likelihood evaluation. More... | |
void | close_streams () |
Helper function that closes the streams from a sampler. | |
Private Member Functions | |
void | initialize_mpi () |
void | initialize_ladder () |
double | update_annealing_params () |
double | find_beta (Interpolator1D &fLambda, int k, double Lambda, double eps=1e-12, int MAX_ITR=1e8) |
void | write_annealing_headers () |
void | run_deo_round (int round, int nlength, int thin, int refresh, int verbosity, bool &even_swap, double avg_lklhd[]) |
void | generate_transitions (int niterations, int start, int finish, int thin, int refresh, int verbosity) |
void | deo_swap (const bool even_swap, const int round, const int swap) |
double | finish_deo_round (int rank, int round, double avg_lklhd[]) |
void | write_state () |
Private Attributes | |
S | _sampler |
likelihood_power_tempered * | _L_tmp |
int | _b |
double | _initial_spacing |
int | _TNum |
int | _E_Num |
int | _ckpt_stride_deo |
bool | _default_cpu_distribution |
bool | _no_initial_ladder |
bool | _write_all |
std::vector< double > | _initial |
int | _current_round |
int | _swap_stride |
int | _current_length |
bool | _restart |
std::vector< double > | _beta |
std::vector< double > | _R |
MPI_Comm | E_COMM |
MPI_Comm | T_COMM |
int | T_size |
int | T_rank |
int | E_size |
int | E_rank |
MPI_Status | Stat |
MPI_Status | Stat2 |
MPI_Request | Req |
MPI_Request | Req2 |
std::string | _old_annealing_file |
std::string | _anneal_file |
std::string | _anneal_summary_file |
std::ofstream | _anneal_out |
std::ofstream | _anneal_summary_out |
Additional Inherited Members | |
![]() | |
virtual void | write_chain_header () |
virtual void | write_state_header () |
![]() | |
int | _seed |
Ran2RNG | _rng |
std::vector< std::string > | _var_names |
size_t | _dimension |
size_t | _step_count |
likelihood * | _L |
std::string | _chain_file |
std::string | _state_file |
std::string | _sampler_file |
std::string | _ckpt_file |
std::ofstream | _chain_out |
std::ofstream | _state_out |
std::ofstream | _sampler_out |
int | _output_precision |
int | _ckpt_stride |
double | _sum_lklhd |
MPI_Comm | _comm |
int | _rank |
Detailed Description
template<class S>
class Themis::sampler_deo_tempering_MCMC< S >
Runs a parallel tempered mcmc chain using a exploration kernel based on the sampler_exploration_mcmc_base class. This uses the parallel tempering scheme from Syed et al. (2020), which is based on the deterministic even-odd swapping scheme, which is a non-reversible markov chain. For more information on the tempering please see Syed 2020).
Member Function Documentation
double estimate_bayesian_evidence | ( | double | avg_lklhd[], |
double | beta[] | ||
) |
- Parameters
-
avg_lklhd[] Vector of likelihood expectations to compute the evidence, using trapezoid rule. beta[] Temperatures of a run.
- Note
- Currently does not work! TODO.
|
inlinevirtual |
- Parameters
-
chain_file The file name of the chain. state_file The file name of the saved state.
- Note
- if no chain_file or state_file is provided it will just read in the one that was saved using set_output_stream. This also assumes that the first element of the state vector is the log-likelihood value.
Reimplemented from sampler_MCMC_base.
|
inlinevirtual |
- Note
- Only run this after calling run sampler.
Reimplemented from sampler_MCMC_base.
|
inlinevirtual |
- Parameters
-
ckpt_in A reference to the input stream.
Implements sampler_MCMC_base.
|
virtual |
- Parameters
-
ckpt_file The file name of the checkpoint file.
Reimplemented from sampler_MCMC_base.
void read_initial_ladder | ( | std::string | annealing_file | ) |
- Parameters
-
annealing_file is the file containing the annealing information for the run. Namely the round, ladder, and rejection rates.
|
virtual |
- Parameters
-
number_of_rounds How many rounds you want to run the sampler for. You can run this many times to build up rounds. thin The thinning rate of the chain. Every thin'd step will be saved. refresh How often cout is updated. If < 0 it is never updated. verbosity If 1 it will save the chain information for all tempering levels.
- Note
- The total number of samples will be given by start_samples*swap_stride.
Implements sampler_MCMC_base.
void set_annealing_schedule | ( | double | initial_spacing, |
int | geometric_increase = 2 |
||
) |
- Parameters
-
initial_spacing The initial geometric spacing for the ladder. That is, the inverse temperature for the ith rung will be given by 1.0/geometric_increase^i. geometric_increase The geometric increase for the number of steps to be used for each round. This should almost always be 2.
void set_cpu_distribution | ( | int | num_temperatures, |
int | num_exploration | ||
) |
- Parameters
-
num_temperatures The number of temperatures for the sampler. num_exploration The number of processors to send each exploration sampler.
- Note
- If this isn't called we use the default distribution which set num_temperatures equal to the number of MPI instances, and num_exploration to 1.
void set_deo_round_params | ( | int | initial_length, |
int | swap_stride | ||
) |
- Parameters
-
initial_length The number of swaps to do in the first round.. swap_stride The number of steps to take before we swap temperatures. Typically this should be at least 5.
- Note
- The default is 10 swaps initially and a swap stride of 25. This is probably too short.
|
virtual |
- Note
- For DEO every chain,state,sampler file will be prefixed with roundXXX_ to denote which deo round it is on.
Reimplemented from sampler_MCMC_base.
The documentation for this class was generated from the following file:
- sampling/sampler_deo_tempering_MCMC.h