Defines the interface for models that generate movie data with a collection of utility functions that allow the user to compute visibility amplitudes, closure phases, etc. Also will accept a movie interpolator function that allows the user to specify how the frame of the movie is chosen. More...
#include "model/model_movie.h"
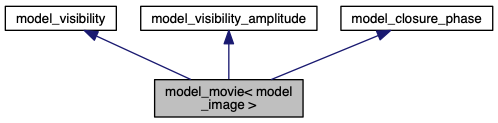
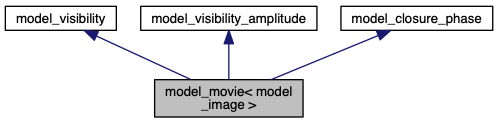
Public Member Functions | |
model_movie (std::vector< double > observation_times) | |
virtual size_t | size () const |
A user-supplied function that returns the number of the parameters the model expects. | |
virtual void | generate_model (std::vector< double > parameters) |
A user-supplied one-time generate function that permits model construction prior to calling the closure_phase for each datum. Takes a vector of parameters. | |
virtual std::complex< double > | visibility (datum_visibility &d, double accuracy) |
Returns visibility in Jy, computed numerically. The accuracy parameters isn't used presently. | |
virtual double | visibility_amplitude (datum_visibility_amplitude &d, double accuracy) |
Returns visibility amplitude in Jy, computed numerically. The accuracy parameters isn't used presently. | |
virtual double | closure_phase (datum_closure_phase &d, double accuracy) |
Returns closure ampitudes, computed numerically. The accuracy parameter is not used at present. | |
void | get_movie_frame (double t_frame, std::vector< std::vector< double > > &alpha, std::vector< std::vector< double > > &beta, std::vector< std::vector< double > > &I) const |
Provides direct access to the constructed image. Sets a 2D grid of angles (alpha, beta) in radians and intensities in Jy per pixel. | |
void | get_visibilities (std::vector< std::vector< double > > &u, std::vector< std::vector< double > > &v, std::vector< std::vector< std::complex< double > > > &V) const |
Provides direct access to the complex visibilities. Sets a 2D grid of baselines (u,v) in lambda, and visibilites in Jy. | |
void | get_visibility_amplitudes (std::vector< std::vector< double > > &u, std::vector< std::vector< double > > &v, std::vector< std::vector< double > > &V) const |
Provides direct access to the visibility amplitudes. Sets a 2D grid of baselines (u,v) in lambda, and visibilites in Jy. | |
void | output_movie_frame (double t_frame, std::string fname, bool rotate=false) |
Outputs the frame of the movie at time t to the file with name fname. If rotate is true then each frame will be rotated by the position angle. | |
void | use_spline_interp (bool use_spline) |
Provides ability to use bicubic spline interpolator (true) instead of regular bicubic. Code defaults to false. | |
std::vector< double > | get_frame_times () |
Provides the ability to get frame times in the movie. | |
virtual void | set_mpi_communicator (MPI_Comm comm) |
Defines a set of processors provided to the model for parallel computation via an MPI communicator. Only facilates code parallelization if the model computation is parallelized via MPI. | |
model_image * | get_movie_model_frame (size_t i) |
Provides access to the the individual model_image frames of the base movie. Requires the movie index. | |
Protected Member Functions | |
virtual size_t | find_time_index (double tobs) const |
Protected Attributes | |
bool | _generated_model |
std::vector< double > | _observation_times |
std::vector< model_image * > | _movie_frames |
std::vector< double > | _parameters |
![]() | |
MPI_Comm | _comm |
![]() | |
MPI_Comm | _comm |
![]() | |
MPI_Comm | _comm |
Detailed Description
template<class model_image>
class Themis::model_movie< model_image >
/details While many EHT models provide images time variability of thus movies are important when understanding accretion flow and spacetime dynamics. This class provides the general interphase for making movies whose models the are inherently time dependent, such as the model_image_orbiting_spot.h from Broderick & Loeb 2006. This is accomplished my creating a series of model_image.h clases at the times specified by the user. Like the model image class this class provides both an interface to interferometric data models and utility functions for computing the appropriate data types. Furthermore, the model expects the last parameter given to be the rotation agle of the image on the sky.
- Warning
- This class contains multiple virtual functions making it impossible to generate and explicit instantiation. This also relies on the model_image.h functionality to compute the FFTW to generate the compex visibilities.
The documentation for this class was generated from the following file:
- model/model_movie.h