Defines a likelihood that non-linearly optimizes over gain corrections with a Gaussian prior. More...
#include "likelihood/likelihood_optimal_complex_gain_visibility.h"
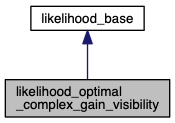
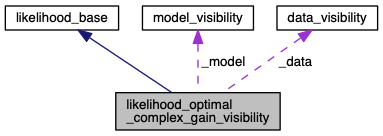
Public Member Functions | |
likelihood_optimal_complex_gain_visibility (data_visibility &data, model_visibility &model, std::vector< std::string > station_codes, std::vector< double > sigma_g) | |
Construct to do scan-by-scan correction by default. | |
likelihood_optimal_complex_gain_visibility (data_visibility &data, model_visibility &model, std::vector< std::string > station_codes, std::vector< double > sigma_g, std::vector< double > t_ge) | |
Specify the times of the gain-correction epochs by hand. | |
likelihood_optimal_complex_gain_visibility (data_visibility &data, model_visibility &model, std::vector< std::string > station_codes, std::vector< double > sigma_g, std::vector< double > t_ge, std::vector< double > max_g) | |
Specify the times of the gain-correction epochs by hand. | |
virtual double | operator() (std::vector< double > &x) |
Returns the log-likelihood of a vector of parameters \( \mathbf{x} \). | |
virtual std::vector< double > | gradient (std::vector< double > &x, prior &Pr) |
virtual double | chi_squared (std::vector< double > &x) |
Returns the \( \chi^2 \) of a vector of parameters \( \mathbf{x} \). | |
std::vector< double > | get_gain_times () |
Returns the start time of each gain-correction epoch. | |
std::vector< std::vector< std::complex< double > > > | get_gains () |
Returns the set of gain corrections for each epoch. | |
void | output_gains (std::ostream &out) |
Outputs the set of complex gains in a structure fashion to an output stream. | |
void | output_gains (std::string outname) |
Outputs the set of complex gains in a structure fashion to a file. | |
void | output_gain_corrections (std::ostream &out) |
Outputs the set of gain corrections in a structure fashion to an output stream. | |
void | output_gain_corrections (std::string outname) |
Outputs the set of gain corrections in a structure fashion to a file. | |
virtual void | set_mpi_communicator (MPI_Comm comm) |
Defines a set of processors provided to the model for parallel computation via an MPI communicator. Only facilates code parallelization if the model computation is parallelized via MPI. | |
size_t | number_of_independent_gains () |
Returns the number of independent gains, which is generally less than product of the number of stations and number of epochs. | |
void | set_iteration_limit (int itermax) |
Set the maximum number of iterations in the likelihood maximizer. More iterations results in higher accuracy for the gains, but at the expense of more time. | |
void | read_gain_file (std::string gain_file_name) |
Reads gain file. | |
void | solve_for_gains () |
Turns on gain solver (default is on) | |
void | fix_gains () |
Fixes the gains to last value. | |
void | fix_gains_during_gradient () |
Fixes the gains during gradient evaluation. | |
void | solve_for_gains_during_gradient () |
Fixes the gains during gradient evaluation. | |
void | use_prior_gain_solutions () |
Assume that the previous solutions for the complex gains yield an approximate solution for the next iteration. Note that this does make subsequent gain solves dependent on the prior history. However, it can also make the solution of the gains much faster. It overrides assume_smoothly_varying_gains() and assume_independently_varying gains(). | |
void | assume_smoothly_varying_gains () |
Assume that the gain corrections are correlated, yielding an approximate solution for subsequent times (default). | |
void | assume_independently_varying_gains () |
Assume that the gain corrections are not correlated. | |
![]() | |
void | output_model_data_comparison (std::ostream &out) |
void | output_model_data_comparison (std::string filename) |
Protected Member Functions | |
virtual void | output (std::ostream &out) |
Private Attributes | |
data_visibility & | _data |
model_visibility & | _model |
std::vector< std::string > | _station_codes |
std::vector< double > | _sigma_g |
std::vector< double > | _max_g |
std::vector< double > | _tge |
std::vector< std::vector< std::complex< double > > > | _G |
std::vector< double > | _sqrt_detC |
bool | _use_prior_gain_solutions |
bool | _smoothly_varying_gains |
bool | _solve_for_gains |
bool | _solve_for_gains_during_gradient |
std::vector< std::vector< size_t > > | _datum_index_list |
std::vector< std::vector< std::complex< double > > > | _y_list |
std::vector< std::vector< size_t > > | _is1_list |
std::vector< std::vector< size_t > > | _is2_list |
double | _opi2 |
int | _itermax |
double * | _ogc_y |
double * | _ogc_yb |
size_t * | _ogc_is1 |
size_t * | _ogc_is2 |
double | _mrq_ochisq |
double * | _mrq_atry |
double * | _mrq_beta |
double * | _mrq_da |
double ** | _mrq_oneda |
Additional Inherited Members | |
![]() | |
MPI_Comm | _comm |
Detailed Description
This class takes a visibility data object and a visibility model object, and then returns the log likelihood after minimalization of the log-likelihood within the complex gain-correction sub-space. That is, it sets the model to \( V_{AB}(G_A,G_B;p) = G_A G_B \bar{V}_{AB}(p) \), and numerically maximizes the likelihood independently for each observation epoch, assuming Gaussian priors on the \(|G_A|\) and Gaussian errors. An approximation of the marginalized likelihood is generated using the covariance of the likelihood, though this makes little difference in practice. Gain-reconstruction epochs may be specified explicitly; by default the corrections will be performed by scan.
- Warning
Member Function Documentation
|
virtual |
Returns the gradient of the log-likelihood of a vector of parameters \( \mathbf{x} \) The prior permits parameter checking if required during likelihood gradient evaluation, through the gradients of the prior is applied elsewhere.
Reimplemented from likelihood_base.
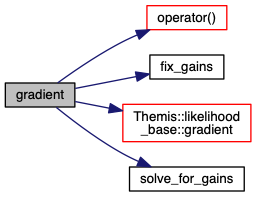
|
protectedvirtual |
Outputs the data and model, as modified by the likelihood appropriately, to the specified output stream. Useful for comparison later.
Reimplemented from likelihood_base.
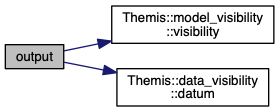
The documentation for this class was generated from the following files:
- likelihood/likelihood_optimal_complex_gain_visibility.h
- likelihood/likelihood_optimal_complex_gain_visibility.cpp